Table of Contents
1What is the concept of AOP? Which problem does it solve?
Aspect Oriented Programming (alias AOP) is a concept of separating cross-cutting parts of an application. For example suppose you want to add logging or some security functions to different elements of your application. Usually you would add some code to each element you want to add that functionality to. But using AOP you can externalize/separate these functions into something called aspects.
2What is a pointcut, a join point, an advice, an aspect, weaving?
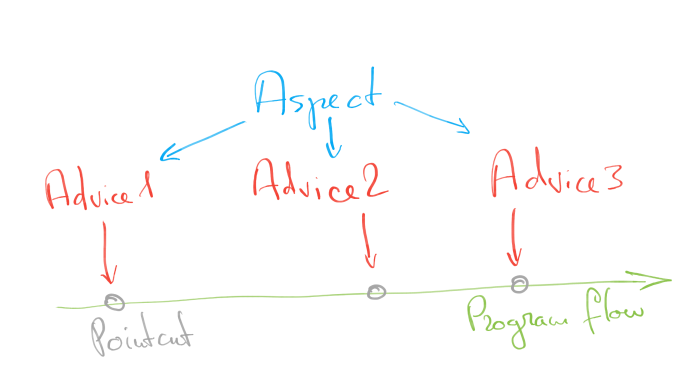
- Pointcut – the exact place where you want to apply the aspect.
- Join point – any possible place that some aspect can be applied to.
- Advice – action of an aspect at a certain join point
- Aspect – defines what advice will be applied at what pointcut
- Weaving – applying aspects on target objects and obtaining some proxied objects.
3How does Spring solve (implement) a cross cutting concern?
In comparison to some AOP frameworks like AspectJ, Spring allows for aspects to be applied only at methods join points (AspectJ can advise fields, types etc.).
Spring AOP is done using proxy objects that in their turn are created by 2 different means:
- JDK dynamic proxying (creating some class that implements the same interface as the proxied bean)
- CGLib proxying (creating subclass for the proxied bean)
important to mention here is the fact that starting from version 3.2 you don’t have to attach any additional libraries to support CGLib proxying.
4Which are the limitations of the two proxy-types?
Common limitations:
- Proxying works only when calls to methods are beyond the limits of a bean. If one method calls another on internally in a bean – that call will not be intercepted.
- Proxied objects must be created by the Spring IoC container.
- Proxies are not serializable.
JDK dynamic proxying has the following limitations:
- can be applied only on objects that implement an interface
CGLib proxying limitations:
- final methods cannot be advised (remember you have to create a subclass for the proxied object so it won’t be possible because of the final modifier)
- the proxied class must have a default constructor
- resulting proxy object is final – you won’t be able to proxy a proxy
5How many advice types does Spring support. What are they used for?
Depending on when the advice is applied there can be the following advice types:
- Before – executes just before the join point
- After – executes after the join point (it doesn’t matter whether the join point run smoothly or threw an exception)
- Around – the moment for the joint point to run can be specified manually in this type of advice, moreover you may choose to skip the method execution or just throw some exception without running the advised method.
- After returning – will be executed if the advised method completes normally
- After throwing – will be executed if the advised method throws some exception
Although around covers most of the cases you need Spring authors advise us to use the least powerful advice type that fulfills our requirements.
6What do you have to do to enable the detection of the @Aspect annotation?
Depending on the container configuration type you will need the following in order to enable @Aspect annotation:
- in Java configuration: add @EnableAspectJAutoProxy annotation to the @Configuration annotated class
- in XML: add <aop:aspectj-autoproxy/> (will require the aop namespace added to the <bean> tag)
7Name three typical cross cutting concerns
- Logging
- Security
- Monitoring
8What two problems arise if you don’t solve a cross cutting concern via AOP?
- Brittle object hierarchy – when seemingly safe modification of superclass causes subclasses to malfunction.
- Cumbersome delegation – you call one thing but that thing doesn’t do the job … instead it asks some 3rd party to do the required job.
9What does @EnableAspectJAutoProxy do?
@EnableAspectJAutoProxy is added to any @Configuration annotated class and does the same thing that <aop:aspectj-autoproxy/> does – enables @Aspect usage.
It may have an additional parameter – proxyTargetClass=true will cause the enforced usage of CGLib.
10What is a named pointcut?
A named pointcut is just a pointcut that is separated from the advice and can be used by many advices.
11How do you externalize pointcuts? What is the advantage of doing this?
Externalizing is done as follows:
It is declared using @Pointcut over a void returning method.
@Pointcut("execution(* someMethod(..))") public void ourFirstPointcut(){} @Before("ourFirstPointcut()") //we call our named pointcut public void doSomethingBefore(){ //actions to be performed before any method whose name is someMethod() }
The advantage is that you can use the same pointcut for different advices and if changes are required you will have to do them in 1 single place.
12What is the JoinPoint argument used for?
It is used for retrieving different information about the advised method (if talking about Spring AOP particularly).
Here is an example that prints the list of the parameters of the advised method:
@Before("execution(* printSomething(..))") public void fromTheAspect(JoinPoint jp) { MethodSignature signature = (MethodSignature) jp.getSignature(); System.out.println("From the @Before of the aspect, advised method parameters: " + Arrays.asList(signature.getParameterNames())); }
13What is a ProceedingJoinPoint?
ProceedingJoinPoint is used to reference the method that is being advised with an @Around advice. in the method that defines the actions to be taken during the around advice you need to call the ProceedingJoinPoint’s proceed() method for the advised method to run.
package blog.codingideas.aspects; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.springframework.stereotype.Component; @Component @Aspect public class CustomAspect { @Pointcut("execution(* printSomething(..))") private void pointcut(){} @Around("pointcut()") public void aroundAdvice(ProceedingJoinPoint pjp) throws Throwable { System.out.println("Before"); // will print "Before" before the execution of printSomething() pjp.proceed(); System.out.println("After"); } }
14What are the five advice types called?
- Before
- After
- Around
- AfterReturning
- AfterThrowing
15Which advice do you have to use if you would like to try and catch exceptions?
You have to use @AfterThrowing with 2 attributes:
- pointcut (alias value) – for selecting a named pointcut or writing an inline one
- throwing – for choosing a name to be passed to the advice method. This name must match the name of the parameter of the @AfterThrowing annotated method.
package blog.codingideas.aspects; import org.aspectj.lang.annotation.AfterThrowing; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.springframework.stereotype.Component; @Component @Aspect public class CustomAspect { @Pointcut("execution(* throwAnException(..))") private void pointcutForException() { } @AfterThrowing(pointcut = "pointcutForException()", throwing = "exception") public void adviceForExceptionThrowing(Exception exception) { System.out.println("###### " + exception.getMessage() + " ######"); } }
16What is the difference between @EnableAspectJAutoProxy and <aop:aspectj-autoproxy>?(this is a question from Core Spring 4.2-4.3 Certification Study Guide
17 Additional resources
If you’re interested to better understand AOP and compare its usage across different platforms, please check an article by MARKO PAĐEN from Toptal:
https://www.toptal.com/dot-net/aspect-oriented-programming-tutorial